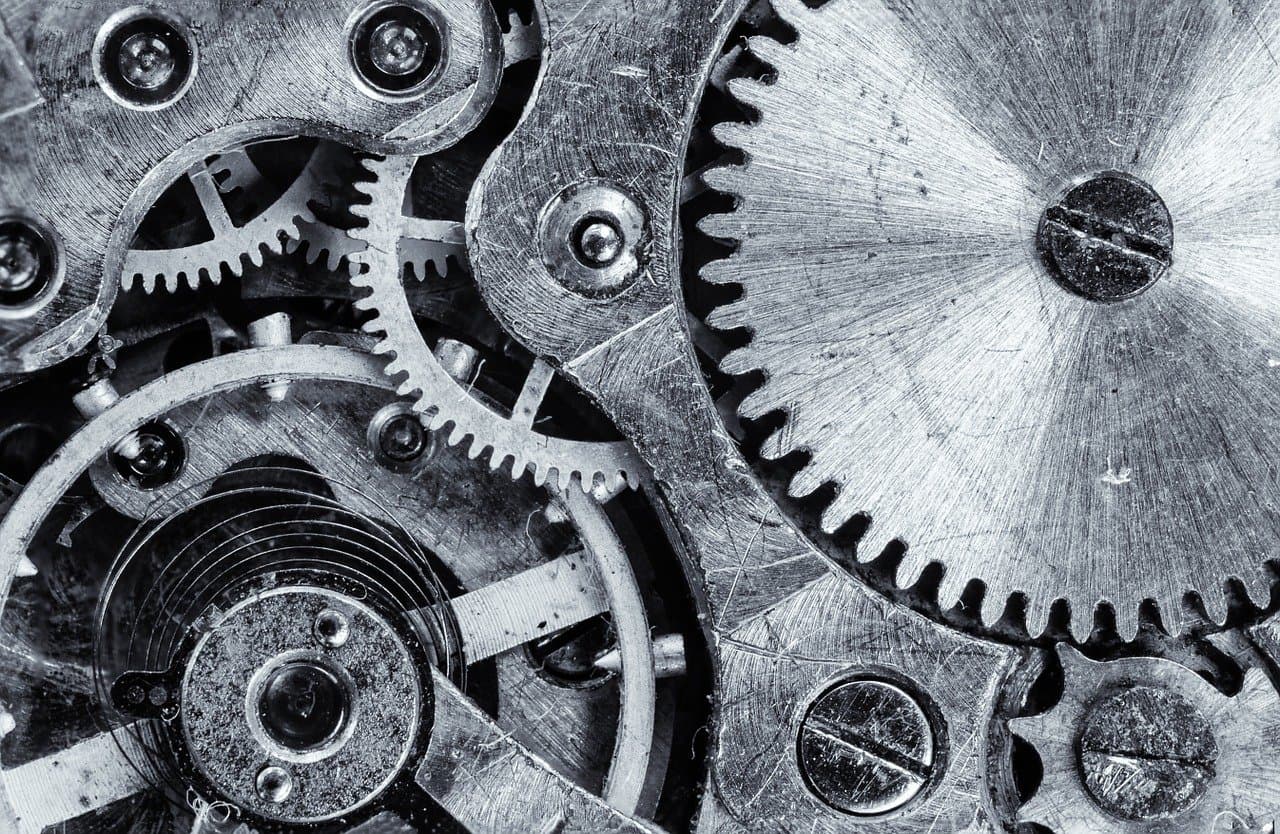
- Published on
Automating Manufacturing Processes with Python
As a Manufacturing Engineer at Applied Materials, I encountered a common challenge that many in the semiconductor industry face: managing complex Bills of Materials (BOMs) during technology transitions. This process was not only time-consuming but also prone to human error. That's when I decided to leverage Python to create a solution that would transform our workflow.
The Challenge
Our team was spending countless hours manually reviewing BOM changes, with over 50 users across the U.S. and India needing to analyze hundreds of nested component changes during technology transitions. The existing process relied heavily on Excel spreadsheets, which were becoming increasingly unwieldy as our operations scaled.
The Solution
I developed a Python GUI application using Tkinter that revolutionized how we handle BOM analysis. Here's how we built it:
1. User Interface Design
- Created an intuitive interface that allowed users to easily upload and compare BOMs
- Implemented filtering capabilities for quick data analysis
- Added visual indicators for critical changes
2. Data Processing
- Developed algorithms for efficient comparison of nested components
- Implemented linear-time filtering to handle large datasets
- Created a robust error handling system
3. Database Integration
- Designed a MySQL database to replace slow-loading Excel files
- Implemented efficient querying for sub-second response times
- Created a caching system for frequently accessed data
The Impact
The implementation of this solution led to significant improvements:
- Reduced manual data sifting time by 500+ man-hours per week
- Improved accuracy in identifying part changes
- Enabled faster decision-making in supply chain resolutions
- Streamlined communication with key customers like Intel and TSMC
Technical Implementation
Here's a simplified example of how we handled the BOM comparison logic:
def compare_boms(original_bom, new_bom):
differences = {}
for part_number in original_bom:
if part_number in new_bom:
if original_bom[part_number] != new_bom[part_number]:
differences[part_number] = {
'original': original_bom[part_number],
'new': new_bom[part_number],
'type': 'modified'
}
else:
differences[part_number] = {
'original': original_bom[part_number],
'new': None,
'type': 'removed'
}
for part_number in new_bom:
if part_number not in original_bom:
differences[part_number] = {
'original': None,
'new': new_bom[part_number],
'type': 'added'
}
return differences